main.dart
import 'package:flutter/material.dart';
void main() => runApp(const FlutterExample());
class FlutterExample extends StatelessWidget {
const FlutterExample({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Example',
theme: ThemeData(primarySwatch: Colors.orange),
home: const StateExample()
);
}
}
class StateExample extends StatefulWidget {
const StateExample({Key? key}) : super(key: key);
@override
_StateExampleState createState() => _StateExampleState();
}
class _StateExampleState extends State<StateExample> {
final PageController controller = PageController(initialPage: 0);
final colors = ["Red", "Green", "Yellow", "Black", "Pink"];
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: const Color(0xFFF5F5F5),
appBar: AppBar(
title: const Text("Flutter - PageView Builder")
),
body: bodyContent()
);
}
bodyContent(){
return PageView.builder(
scrollDirection: Axis.horizontal,
controller: controller,
itemCount: colors.length,
itemBuilder: (context, index){
return (pageContent(colors[index]));
},
);
}
pageContent(String text){
return Container(
color: Colors.blueGrey[100],
child: Center(
child: Text(
text,
style: const TextStyle(
fontSize: 44,
fontWeight: FontWeight.bold
),
),
),
);
}
}
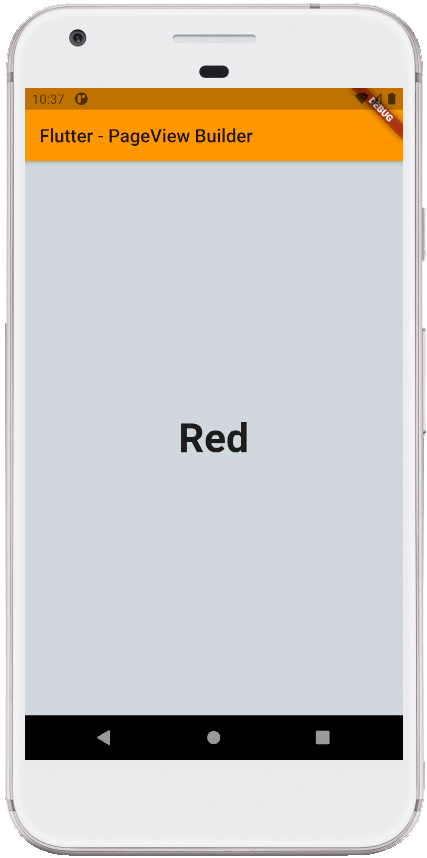
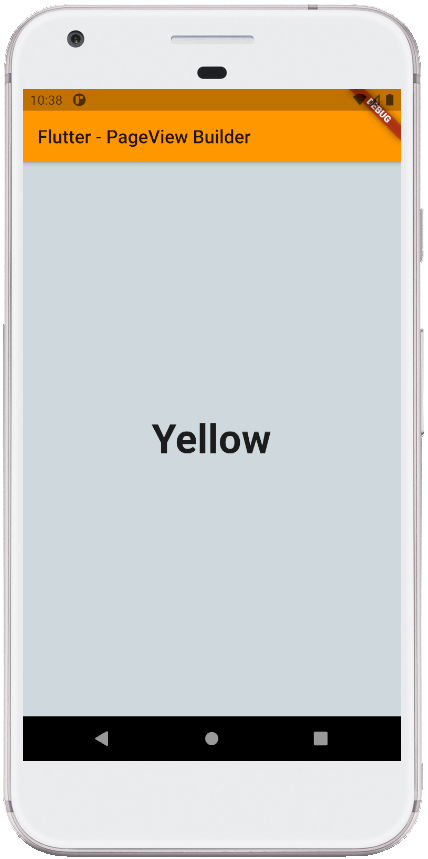
More flutter tutorials
- flutter – AlertDialog example
- flutter – AlertDialog background color
- flutter – AlertDialog width
- flutter – AlertDialog height
- flutter – AlertDialog actions center
- flutter – Expanded example
- flutter – Expanded flex
- flutter – Align exact position
- flutter – Container transform rotation
- flutter – BoxDecoration example
- flutter – Chip example
- flutter – Container circle border
- flutter – Container gradient border
- flutter – How to show hide status bar
- flutter – AppBar center title